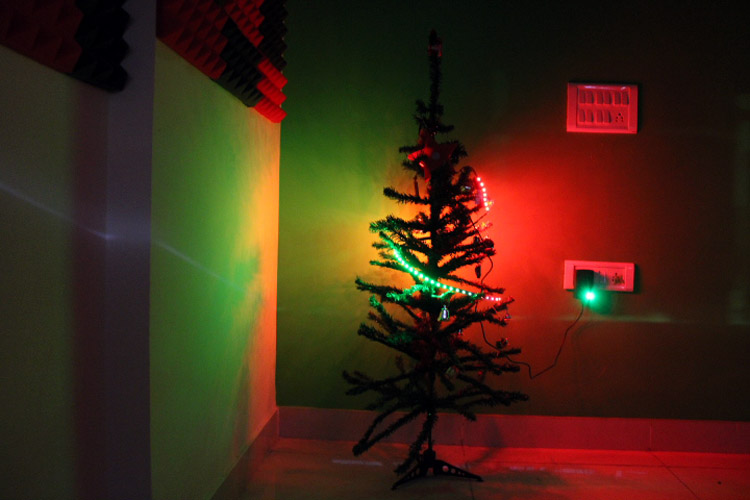
As Christmas is around the corner and the essence of it is incomplete without the decoration and the Christmas tree. So, instead of using the same old Christmas tree ornaments that we put up every year, let's step it up a notch and use Arduino with Neo pixel LED Strip, JQ6500 Voice Module, and PIR Sensor. The PIR sensor is used to detect movement, the JQ6500 Voice Module is used to play the Christmas carol and Neo Pixels are used to light up the Christmas tree.
Components Required
- Arduino Nano
- Neo Pixel LED Strip
- PIR Sensor
- JQ6500 Voice Sound Module
- 8Ω 0.5W Speaker
- 7805 Voltage Regulator
- DC Power Jack (Female)
- 2× 10µf Capacitor
Circuit Diagram
The complete circuit diagram for Arduino Based Decorative Christmas Tree is given above. It is pretty simple as we only need to connect a PIR sensor, JQ6500 voice module, and Neo-Pixel LED Strip. The complete setup is powered by a 12V battery. The brain of the circuit is Arduino Nano. The PIR sensor is used to detect the presence of a person. Two of the three pins of this sensor namely Vcc & GND are connected to Arduino’s 5V and GND. While the data pin is connected to the D5 of Arduino Nano and the data pin of the Neo-Pixel strip is connected to the D2 pin of Arduino Nano.
The JQ5600 MP3 module is a 3.3V logic module, so you can’t connect it directly to the Arduino’s IO pins, but it is fine to be powered from the Arduino’s 5V power line. RX and TX pins of the MP3 module are connected to digital pin 9 and 8 of the Arduino Nano. A 1kΩ resistor is put in between the Arduino digital pin 9 and MP3 module's RX to drop the voltage down from the Arduino’s 5V.
Programming Arduino Nano for Decorative Christmas Tree
Once we are ready with our hardware, we can connect the Arduino to our Computer and start programming. The complete code for this project is given at the bottom of this page; you can upload it directly to your Arduino board. However, if you are curious to know how the code works read further.
The code uses JQ6500_Serial.h, Adafruit_NeoPixel.h and SoftwareSerial.h libraries. SoftwareSerial library comes pre-install with Arduino IDE. The JQ6500 Serial library can be installed from this link. While Adafruit_NeoPixel.h library can be directly downloaded from Arduino IDE. For that, go to the Sketch > Include Library > Manage Libraries. Then search for ‘Adafruit Neo’ in the search box and download and install the ‘Adafruit Neopixel’ library.
As usual start the code by including all the required libraries and defining all the pins used in this project.
#include <Arduino.h> #include <SoftwareSerial.h> #include <JQ6500_Serial.h> #include <Adafruit_NeoPixel.h>
After that, declare the NeoPixel strip object, where Argument 1 is the number of pixels in the NeoPixel strip and Argument 2 is the Arduino pin where the LED strip is connected.
Adafruit_NeoPixel strip(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800);
Then inside the setup() function initialize Input-Output pins and also initialize the serial monitor and JQ6500 voice module.
pinMode(sensor, INPUT); mp3.begin(9600); mp3.reset(); mp3.setVolume(50); mp3.setLoopMode(MP3_LOOP_NONE); strip.begin();
Inside the main loop first, we are starting the rainbow mode of the Neo-Pixel LED strip and then reading the PIR sensor state. Now if the state of the PIR sensor is high we will call the mp3.play() function to play the Merry Christmas song and also change the Neo-Pixel state to theaterChaseRainbow mode.
void loop(){ rainbow1(10); val = digitalRead(sensor); Serial.print(val); if (val == HIGH) { mp3.playFileByIndexNumber(1); theaterChaseRainbow(50); delay(7000); } }
3D Printing Star-shaped Enclosure
Next, I measured the dimensions of the setup using the scale and also measured the dimensions of the barrel jack to design a casing for my setup. My design looked something like this below once it was done.
After finishing the design, I exported it as an STL file, sliced it based on printer settings, and finally printed it. The STL file is also available for download from Thingiverse and you can print your casing using it. After the print was done, I proceeded with assembling the project set up in a permanent enclosure to install it on the Christmas tree. With the complete connection made I assembled the circuit into my casing and everything was a nice fit as you can see here.
Christmas Star Testing
Once the code and hardware are ready, upload the code on Arduino Nano. After that fit everything inside the enclosure as shown below image:
Now, power the setup using a 12V adapter, Neopixel will start illuminating in rainbow mode, and when the PIR sensor detects a person it will play the ‘Merry Christmas’ song and also change the Neo-Pixel mode from rainbow to Theaterchase.
#include <Arduino.h> #include <SoftwareSerial.h> #include <JQ6500_Serial.h> #include <Adafruit_NeoPixel.h> JQ6500_Serial mp3(8,9); #define PIXEL_PIN 2 // Digital IO pin connected to the NeoPixels. #define PIXEL_COUNT 55 // Number of NeoPixels Adafruit_NeoPixel strip(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800); const int sensor = 5; const int state = LOW; int val; int mode = 0; // Currently-active animation mode, 0-9 int r,g,b,data; void setup() { Serial.begin(115200); pinMode(sensor, INPUT); mp3.begin(9600); mp3.reset(); mp3.setVolume(50); mp3.setLoopMode(MP3_LOOP_NONE); strip.begin(); // Initialize NeoPixel strip object (REQUIRED) strip.show(); // Initialize all pixels to 'off' } void loop(){ val = digitalRead(sensor); Serial.print(val); if (val == HIGH) { mp3.playFileByIndexNumber(1); // theaterChaseRainbow(5); delay(9000); Serial.print("Working"); } rainbow(); } void rainbow() { for(long firstPixelHue = 0; firstPixelHue < 3*65536; firstPixelHue += 256) { for(int i=0; i<strip.numPixels(); i++) { // For each pixel in strip... int pixelHue = firstPixelHue + (i * 65536L / strip.numPixels()); strip.setPixelColor(i, strip.gamma32(strip.ColorHSV(pixelHue))); } strip.show(); // Update strip with new contents //delay(wait); // Pause for a moment } } /*void theaterChaseRainbow() { int firstPixelHue = 0; // First pixel starts at red (hue 0) for(int a=0; a<30; a++) { // Repeat 30 times... for(int b=0; b<3; b++) { // 'b' counts from 0 to 2... strip.clear(); // Set all pixels in RAM to 0 (off) for(int c=b; c<strip.numPixels(); c += 3) { int hue = firstPixelHue + c * 65536L / strip.numPixels(); uint32_t color = strip.gamma32(strip.ColorHSV(hue)); // hue -> RGB strip.setPixelColor(c, color); // Set pixel 'c' to value 'color' } strip.show(); // Update strip with new contents //delay(wait); // Pause for a moment firstPixelHue += 65536 / 90; // One cycle of color wheel over 90 frames } } }*/