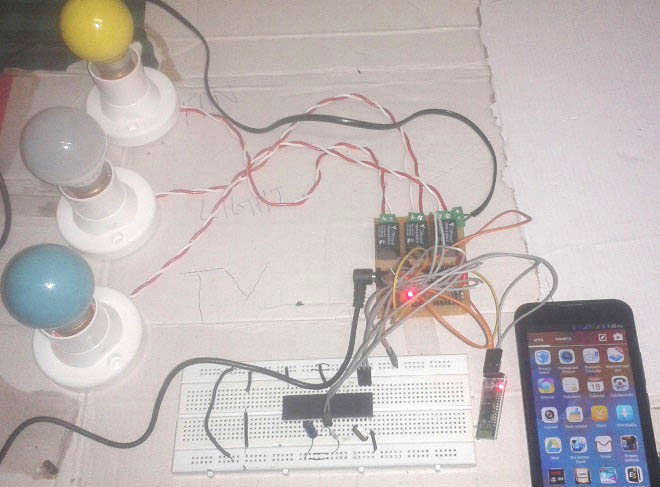
Imagine that you can control the electronic appliances of your home from anywhere inside the house, just using your Smart phone. In this project, we will use wireless Bluetooth technology to control the Home Electronic Appliances through a Android Phone. Bluetooth has a range of 10-15 meters, so that you can switch ON and OFF any electronic appliance within the range. We have also developed a Toy car controlled by Android Phone, using Bluetooth module and Arduino.
Here we have used 8051 microcontroller with a Bluetooth module, for wirelessly receive the data, sent from the Android Phone. So that microcontroller can Turn ON and OFF the home appliances accordingly. [Check here more 8051 microcontroller based projects]
Main Components
- 8051 microcontroller
- Bluetooth Module HC05
- Relay
- ULN2003
- Bulb
- Holder
- Wire
- IC 7805
- Android phone
- Bluetooth controller app Android app
- 10uf capacitor
- 1000uf capacitor
- 10K resistor
- 1k resistor
- Power Supply
Bluetooth Module:
HC-05 Bluetooth module consists two things one is Bluetooth serial interface module and a Bluetooth adaptor. Bluetooth serial module is used for converting serial port to Bluetooth.
How to operate Bluetooth module?
You can directly use the Bluetooth module after purchasing from market, because there is no need to change any setting of Bluetooth module. Default baud rate of new Bluetooth module is 9600 bps. You just need to connect rx and tx to controller or serial converter and give 5 volt dc regulated power supply to module.
Bluetooth module has two modes one is master mode and second one is slave mode. User can set either mode by using some AT commands. Even user can set module’s setting by using AT command. Here is some commands uses are given:
First of all user need to enter AT mode with 38400 bps baud rate by pressing EN button at Bluetooth module or by giving HIGH level at EN pin. Note: all commands should ends with \r\n (0x0d and 0x0a) or ENTER KEY from keyboard.
After it if you send AT to module then module will respond with OK
AT → Test Command
AT+ROLE=0 → Slave Mode select
AT+ROLE=1 → Master Mode select
AT+NAME=xyz → Set Bluetooth Name
AT+PSWD=xyz → Set Password
AT+UART=<value1>,<value2>,<value3> → set Baud rate
Eg. AT+UART=9600,0,0
Pin Description of accelerometer:
- STATE → Open
- Rx → Serial receiving pin
- Tx → Serial transmitting pin
- GND → ground
- Vcc → +5volt dc
- EN → to enter in AT mode
Working Explanation:
In this project we have used 8051 microcontroller for controlling the whole process of this project. And a Bluetooth module is used for controlling the home appliances wirelessly. Home appliances will turned ON and OFF when user will touch button in the Bluetooth mobile app in Android mobile phone. To run this project, first we need to download Bluetooth app form Google play store. We can use any Bluetooth app that can send data using Bluetooth. Here are some apps name that can be used:
- Bluetooth Spp pro
- Bluetooth controller
After installing the App, you need to open it and then search Bluetooth device and select HC-05 Bluetooth device. And then configure keys.
Here in this project we have used Bluetooth controller app.
- Download and install Bluetooth Controller.
- Turned ON mobile Bluetooth.
- Now open Bluetooth controller app
- Press scan
- Select desired Bluetooth device (Bluetooth Module HC-05).
- Now set keys by pressing set buttons on screen
To set keys we need to press ‘set button’ and set key according to picture given below:
After setting keys press ok.
You can see in the above picture that there are 9 buttons in which first row is for fan controlling, second one is for light controlling and last one is for TV controlling. Means First row’s ON and OFF buttons are used to ON and OFF the fan, second row’s buttons are for Light and third ones are for TV. We have used three bulbs of different colors instead of TV and fan, for demonstration purpose.
Now, when we touch any button in Bluetooth controller app then Android phone sends a value to Bluetooth module, after receiving this value, Bluetooth module sends the received value to the microcontroller and then microcontroller reads it and compare it with predefined value. If any match is occurred then microcontroller performs relative operation. Same operation will performed each time when button pressed.
Now, when user touch ‘Fan On’ button in Bluetooth controller app then microcontroller receives ‘1’ via Bluetooth module and then controller Switch ‘On’ the Fan by using relay driver and relay. And when user touch ‘Fan Off’ button in Bluetooth controller app then microcontroller receives ‘2’ via Bluetooth module and then controller Switch ‘Off’ the Fan by using relay driver and relay.
Likewise 3,4,5,6 numbers are sent by Android Phone, when Light On, Light Off, TV On, TV Off button has been touched respectively:
Button |
Data |
Operation |
Fan On |
1 |
Fan Turned On |
Fan Off |
2 |
Fan Turned Off |
Light On |
3 |
Light Turned On |
Light Off |
4 |
Light Turned Off |
TV On |
5 |
TV Turned On |
TV Off |
6 |
TV Turned Off |
Circuit Diagram and Explanation
Circuit connections of this project are very simple. Bluetooth module’s Rx and Tx pins are directly connected to the Tx and Rx pins of Microcontroller. Three 5 volt relays are used as a switch for turning On and Off the home appliances running on AC mains. And a relay driver ULN2003 is used for driving relays. Fan, Light and TV are connected at P2.1, P2.2 and P2.3 via relays and relay driver. An 11.0592 MHz Crystal oscillator is used in this circuit for generating clock signal for microcontroller. And a 5 volt voltage regulator LM7805 is used for provide 5 volt for the whole circuit.
Program Explanation:
In this program, first of all we have included header file and defines input, output pins and variables.
#include<reg51.h> sbit Fan=P2^0; sbit Light=P2^1; sbit TV=P2^2; char str; char Charin=0;
After this we have created a function for delay.
void delay(int time) { unsigned int i,j; for(i=0;i<time;i++) for(j=0;j<1275;j++); }
Here we have some functions that we have used in our program. In this we have configured 9600bps baud rate at 11.0592MHz Crystal Frequency.
void Serialwrite(char byte) { SBUF=byte; while(!TI); TI=0; } void Serialprintln(char *p) { while(*p) { Serialwrite(*p); p++; } Serialwrite(0x0d); } void Serialbegin() { TMOD=0x20; SCON=0x50; TH1=0xfd; TR1=1; }
After this, in main program we have initialized UART and monitored the SBUF register for receiving the data. Then data is matched and compared with predefined values and relative operation has been performed.
void main() { P2=0x00; Serialbegin(); Serialprintln("System Ready..."); delay(50); while(1) { while(!RI); Charin=SBUF; str=Charin; RI=0; if(str=='1') { Fan=1; Serialprintln(" Fan ON"); delay(50); } else if(str=='2') { Fan=0; Serialprintln(" Fan OFF"); delay(50); }
So that’s how we can create a whole system for the house and can connect all the AC appliances to the 8051 microcontroller using Relays. And this bluetooth controlled home automation system can be operated from a Smart phone.
Complete Project Code
#include<reg51.h>
sbit Fan=P2^0;
sbit Light=P2^1;
sbit TV=P2^2;
char str;
char Charin=0;
void delay(int time)
{
unsigned int i,j;
for(i=0;i<time;i++)
for(j=0;j<1275;j++);
}
void Serialwrite(char byte)
{
SBUF=byte;
while(!TI);
TI=0;
}
void Serialprintln(char *p)
{
while(*p)
{
Serialwrite(*p);
p++;
}
Serialwrite(0x0d);
}
void Serialbegin()
{
TMOD=0x20;
SCON=0x50;
TH1=0xfd;
TR1=1;
}
void main()
{
P2=0x00;
Serialbegin();
Serialprintln("System Ready...");
delay(50);
while(1)
{
while(!RI);
Charin=SBUF;
str=Charin;
RI=0;
if(str=='1')
{
Fan=1;
Serialprintln(" Fan ON");
delay(50);
}
else if(str=='2')
{
Fan=0;
Serialprintln(" Fan OFF");
delay(50);
}
else if(str=='3')
{
Light=1;
Serialprintln(" Light ON");
delay(50);
}
else if(str=='4')
{
Light=0;
Serialprintln(" Light OFF");
delay(50);
}
else if(str=='5')
{
TV=1;
Serialprintln(" TV ON");
delay(50);
}
else if(str=='6')
{
TV=0;
Serialprintln(" TV OFF");
delay(50);
}
str=0;
}
}
Comments
this circuit diagram is draw
this circuit diagram is draw by using dip trace software.
and i think it easy to understand. you need to give some of your concentration on the circuit to understand.
try it again.
sir i'm using egbt-045ms as
sir i'm using egbt-045ms as my bluetooth module. what will be the adjustment?
Thanks for reply
Can i usethe same android app. even i'm using egbt-045ms as my bluetooth module?
Sir , please provide the code
Sir , please provide the code with LCD interface also the modified circuit diagram.
Take help of this and LCD
Take help of this LCD Interfacing with 8051 Microcontroller and try to build.
Bluetooth module
Is RS232 MAX required for bluetooth interfacing with microcontroller?
What is TI
sir i need a help to understand what is TI in the program......if register it is not configured....kindly let me know.
SBUF (serial buffer special
SBUF (serial buffer special function register) is used for serial communication, whenever we want to send any byte to serial device we put that byte in SBUF register, when the complete byte has been sent then TI bit is set by hardware. We need to reset it for sending next byte. It’s a flag that indicates that byte has been sent successfully. TI is the second bit of SCON register.
regarding relay specification
Sir whether we require 12v or5v relay ??
Is there will be no damage by using5v relay??
Plzz give info in detail I m confused???
range extension
as bluetooth range is 10-15 meters so if range extension is possible?
SBUF is the 8051's internal
SBUF is the 8051's internal Register which is used in Serial Communication where as 'str' is just user defined variable.
yes you may use another
yes you may use another bluetooth module with good quality antenna.
i can't understood anything
i can't understood anything of the circuite diagram.
please explain the circuite diagram.
did you got the correct code?
did you got the correct code?.. I am in search of it too...
if I change Bluetooth module
if I change Bluetooth module to extension the rang. then is there program will change?
if I increase the rang of
if I increase the rang of Bluetooth module up to 100 meter so what I shall I do. pleaze told me sir.
100 meter is not possible
100 meter is not possible with HC-05 Bluetooth module, better use GSM Based Home Automation, to control the appliances from anywhere.
if I increase the rang of
if I increase the rang of Bluetooth module up to 100 meter so what I shall I do. pleaze told me sir.
Your question is already
Your question is already answered above, why are you posting it again and again. Your comment is only visible after getting approved, so you wont be able to see it just after posting.
can we use arduino instead of
can we use arduino instead of micro controller
yes, check this one Smart
yes, check this one Smart Phone Controlled Home Automation Using Arduino
do u have an assembly code
do u have an assembly code for this project?
interfacing problem
sir can u help me ,doing bluthooth controlled HA ,but the program is not uploded in targeted MC unit ,i have already chekked that all pheriperal interfaces ande power sources ,i am using friendly board of ours
load control using GSM
SIR, i want to control my load using Gsm, not bluetooth, may it possible.
Yes check this one: GSM Based
Yes check this one: GSM Based Home Automation using Arduino
to control home appliances using arduino and bluetooth module
can u plz provide me the code and circuit diagram to control home appliances using arduino and bluetooth module from android mobile..
electonics and communication
is the above code is assembly language
I need help please
Hello guys please I need help, I've done everything as mentioned in the article but the issue I am facing is that when I press 0n key from my phone the relay only ticks very low sound as if it is not being magnetised properly to switch on or off inside relay please tell me a solution the signal from microntroller are not enough to energise the relay even though I'm using relay driver
Which Relay are you using?
Which Relay are you using? Use 5v Relay.
sir if I make ISP burner then
sir if I make ISP burner then what I shall do. plz send circuit diagram of AT89C51 ISP burner
Electronic and communication
Can you please help me about Bluetooth module
Some difficulty
Sir what oscillator is used in circuit for 11.0592 MHz frequency
Need help for communication with comport to Bluetooth
Dear sir,
I have degitizer, which is communicate data to PC over comport. I am a software developer and need communication with Android over Bluetooth.
Can you please provide the circuit to convert from comport to Bluetooth.
I shall be highly grateful to you.
Thanking you
This may be helpful: Smart
This may be helpful: Smart Phone Controlled Home Automation Using Arduino
sir if I make ISP burner then
sir if I make ISP burner then what I shall do. plz send circuit diagram of AT89C51 ISP burner
type of languge
what is the type of this language
is it c or c++ or java or what ?
About project
Sir in video as shown below relay have u connected IC ULN2003A n if u have connected frm dat have taken one i/p wire r frm relay
Source code is complete, you
Source code is complete, you can use 12Mhz crystal but then you need to change the the frequency in Keil uVision software while uploading the code.
home automation system
Sir, could i use the "5V charger or 9V battery through 7805" to supply relays, HC05 and microcontroller instead of using 12V through 7805 ??
home automation system
Sir, could i use the "5V charger or 9V battery through 7805" to supply relays, HC05 and microcontroller instead of using 12V through 7805 ??
header files in the code
HI, its a nice project, got scope for lots of applications,
why are there no header files for bluetooth and usart in the above code.
could you post it on the wall
Sir, what is the function of
Sir, what is the function of S1 (in the left hand side of the circuit diagram). Can we skip it ?
project is not working
Sir....I did all the connections properly.......I programmed microcontroller properly.............Then why it's not working............Please reply me.....
It worked once......
Hi sr vvvvv nice to your
Hi sr vvvvv nice to your project and code vvv thanks sr ........pls led and sd card with Arduino pls workout this project dr
Bluetooth module interfacing with 8051.
this project works will while controlling relay or dc motor etc, but the message along with the activation of relay or motor does't recieved send by the 8051 mcu. i m using "realterm" & "teraterm" serial terminal.
You cannot use the Bluetooth
You cannot use the Bluetooth module directly on Proteus. But you can use the virtual monitor to check if the code is working as expected.
And yes you can use any alternative application from play store
how to use with virtual terminal
virtuL TERMINL window is not taking any input
Right click on the window and
Right click on the window and enable echo
about bluetooth module
In Pin Description of accelerometer where i have to connect EN pin microcontroller ??
about Bluetooth application
give me the link of Bluetooth controller application ....... because in playstore so many applications are there so which one i have to use i don't know so please give me link....
You can use any bluetooth
You can use any bluetooth application. My personal choice is "Bluetooth Terminal" it works well and should also work for this project
ELECTRICAL AND ELECTRONICS ENGINEERING
I WANT TO BE IMPLEMENTING AUDINO PROJECT
BT r both getting paired, but
BT r both getting paired, but if 1,3 or 5 is sent thru app 'Bluetooth Terminal" no changes is occurred even after connecting LED at MCU output pin 21,22, and 23. Any one can help plaese....
Both the BT is paired, but
Both the BT is paired, but when putting 1,3 or 5 pin 21,22 or 23 is not changed as i put led in that pin. can any body say the cause???????????
Ckt connection as per given n
Ckt connection as per given n code upload is also successful, I connected LEDs at o/p pin 21,22,23 with register 330ohm for each and loaded a app ‘Bluetooth Terminal’in android phone and it paired with hc05 correctly,, but even spite of putting 1,3 or 5 on BT terminal nothing is getting changed on pin 21,22 or 23 ie LEDs not getting ON.
my BT module is same as mentioned HC05(BC417).
anybody can help me the problem is what???
thnx
application related
Sir,
application for Bluetooth controller is not available on play store
Please send me app link
project problme
sir i was follow you all or instructions but my projects its NOT run LED only blinking when i applyde to 5v power supply my all connections its correct my mobaile app HC05 its connected but its not run sir plz can you help me ?
Sir i need Android app code
Sir i need Android app code .app that you used for controlling appliances
SOFTWARE TO BURN
sir i use G-540 TO burn program but i couln't burn it kindly tell me which software is use to burn code
Please I can't really interpret the circuit diagram and what software did you use for the simulation (was it proteus)