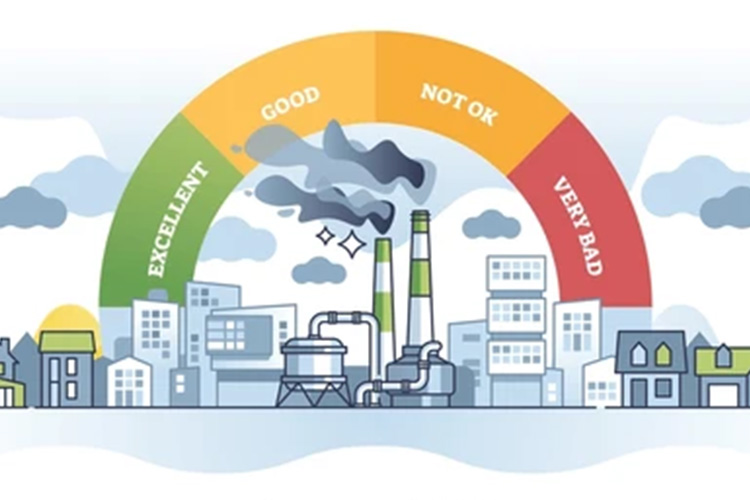
The solution involves developing a hardware device using the Sipeed Maixduino board, equipped with sensors to read pollutant values (such as PM2.5, PM10, O3, CO, etc.). These readings are processed by an onboard MLP (Multi-Layer Perceptron) neural network model, which has been pre-trained to predict the AQI index from the pollutant concentrations. Based on the AQI range, the system provides real-time health suggestions displayed directly on the hardware’s screen. This portable, user-friendly setup enables immediate, actionable feedback, enhancing public awareness and promoting proactive health measures regarding air quality.
To see the full demonstration video, click on the YouTube Video below.
COMPONENTS USED
Working: This LCD uses the I2C communication protocol, which simplifies wiring by using only two data pins (SDA and SCL) to transmit data. It displays characters and numbers in two rows of sixteen columns.
Need: The LCD displays real-time AQI values and predictions. It provides a user-friendly way to view sensor data and AQI category information directly from the device.
2. MQ Sensors (Analog Gas Sensors)
Working: The MQ-7 sensor detects carbon monoxide (CO) in the air. It outputs an analog signal corresponding to the concentration of CO gas.
Need: CO levels are a crucial part of air quality assessment. Monitoring CO helps detect harmful levels of pollution, particularly in indoor and urban environments.
Working: The MQ-135 measures air quality by detecting gases like NH3, NOx, alcohol, benzene, smoke, and CO2. It outputs an analog signal proportional to the gas concentration.
Need: As a versatile air quality sensor, the MQ-135 helps in gauging general pollution levels, essential for estimating the overall AQI.
Working: The MQ-136 detects hydrogen sulfide (H2S) gas, outputting an analog signal related to its concentration.
Need: H2S is a toxic gas often found near industrial areas. Monitoring it helps provide a more comprehensive air quality analysis.
MQ-4 (Methane Sensor):
Working: The MQ-4 detects methane (CH4) gas. It produces an analog signal based on the concentration of methane in the air.
Need: Methane is a flammable gas and a contributor to greenhouse effects. Monitoring its levels is important in both air quality assessment and safety.
MQ-131 (Ozone Sensor):
Working: The MQ-131 sensor detects ozone (O3) gas, providing an analog output proportional to the concentration.
Need: Ozone levels are important for AQI as high ground-level ozone can cause respiratory issues and harm vegetation.
Working: The PMS5003 measures particulate matter (PM) levels, specifically PM2.5, and PM10. It uses laser scattering to detect particle concentration in the air and communicates data via UART (TX/RX pins).
Need: Particulate matter (PM) is a primary factor in calculating AQI. PM2.5 and PM10, in particular, are important indicators of air pollution, as these fine particles can penetrate the respiratory system and impact health.
3. CIRCUIT CONNECTION
LCD (16x2 I2C Display):
Connect the SDA pin to (SDA) on the Maixduino.
Connect the SCL pin to (SCL) on the Maixduino.
Connect VCC to 5V
Connect GND to GND.
MQ Sensors (Analog Sensors):
Connect the MQ-7 (CO Sensor) to A0 (IO33) on the Maixduino.
Connect the MQ-135 (Air Quality Sensor) to A1 (IO32) on the Maixduino.
Connect the MQ-136 (H2S Sensor) to A2 (IO35) on the Maixduino.
Connect the MQ-4 (Methane Sensor) to A3 (IO34) on the Maixduino.
Connect the MQ-131 (Ozone Sensor) to A4 (IO39) on the Maixduino.
For each MQ sensor, connect VCC to 5V and GND to GND on the Maixduino.
Nova PM Sensor (PMS5003):
Connect the TX pin of the PMS5003 to IO5 (TX1) on the Maixduino.
Connect the RX pin of the PMS5003 to IO4 (RX1) on the Maixduino.
Connect VCC to 5V and GND to GND on the Maixduino.
Power Connections:
Connect the VCC of all sensors to the 5V pin on the Maixduino.
Connect the GND of all sensors to the GND pin on the Maixduino.
4.SETTING UP PLATFORMIO IDE
Open Visual Studio Code:
Launch the VS Code application once it's installed.
Install PlatformIO Extension:
In VS Code, go to the Extensions view by clicking on the Extensions icon (usually found on the left sidebar).
Search for "PlatformIO IDE" in the Extensions Marketplace.
Click on "PlatformIO IDE" in the search results, then select "Install."
Open PlatformIO in VS Code:
Once installed, you'll see a PlatformIO icon in the Activity Bar on the left. Click it to open the PlatformIO Home.
Set up your Project:
For Maixduino-based projects, choose the Maixduino board when setting up the project.
After setting up PlatformIO in Visual Studio Code, go to the PlatformIO Home by clicking on the PlatformIO icon in the Activity Bar.
Select Libraries from the PlatformIO toolbar. This will open the Library Manager, where you can search for and download libraries.
Download Libraries Manually:
If you have a library file (for example, from GitHub), you can manually add it.
Download the library as a ZIP file, then unzip it.
Place the unzipped folder in your project directory under .pio/libdeps or directly in the lib folder within your project.
Library Compatibility Check:
PlatformIO ensures compatibility between your libraries and board by showing version compatibility. Make sure the version of the libraries matches the requirements of your Maixduino board.
5. PLATFORM IO (cpp) CODE EXPLANATION
1. Libraries and Initial Setup
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27, 16, 2);
const int mq7Pin = A0; // MQ-7 CO sensor
const int mq135Pin = A1; // MQ-135 Air Quality sensor
const int mq136Pin = A2; // MQ-136 H2S sensor
const int methanePin = A3; // Methane sensor (MQ-4)
const int ozonePin = A4; // Ozone sensor (MQ-131)
#define pmsSerial Serial2
Analog Pins: Defines analog input pins for the gas sensors.
PM Sensor: pmsSerial is assigned to Serial2, used for the Nova PM sensor, which transmits particulate matter data via serial communication.
bool readPMData(int &pm1_0, int &pm2_5, int &pm10);
void setup() {
Serial.begin(115200);
pmsSerial.begin(9600);
lcd.init();
lcd.backlight();
lcd.setCursor(0, 0);
lcd.print("AQI Prediction");
delay(2000);
lcd.clear();
}
2.Loop Function
void loop() {
// Reading analog values from MQ sensors
int mq7Value = analogRead(mq7Pin); // CO level
int mq135Value = analogRead(mq135Pin); // Air quality
int mq136Value = analogRead(mq136Pin); // H2S level
int methaneValue = analogRead(methanePin); // Methane (CH4) level
MQ Sensor Readings: Reads analog values from the MQ sensors to detect the concentration of various gases and stores them in variables mq7Value, mq135Value, mq136Value, and methaneValue.
int pm1_0, pm2_5, pm10;
if (readPMData(pm1_0, pm2_5, pm10)) {
Serial.print("PM 1.0: ");
Serial.println(pm1_0);
Serial.print("PM 2.5: ");
Serial.println(pm2_5);
Serial.print("PM 10: ");
Serial.println(pm10);
}
PM Sensor Readings: Calls readPMData to get the particulate matter values. If the function returns true, it prints PM values (PM 2.5, PM 10) to the Serial Monitor.
Serial.println("------------ Sensor Values ------------");
Serial.print("MQ-7 CO: "); Serial.println(mq7Value);
Serial.print("MQ-135 : "); Serial.println(mq135Value);
Serial.print("MQ-136 H2S: "); Serial.println(mq136Value);
Serial.print("Methane (CH4): "); Serial.println(methaneValue);
Serial Output: Outputs the readings of each MQ sensor to the Serial Monitor for debugging or logging purposes.
// Send data format for the model: "DATA:<mq7Value>,<mq135Value>,<mq136Value>,<pm2_5>,<pm10>,<methaneValue>;"
Serial.print("DATA:");
Serial.print(mq7Value); Serial.print(",");
Serial.print(mq135Value); Serial.print(",");
Serial.print(mq136Value); Serial.print(",");
Serial.print(pm2_5); Serial.print(",");
Serial.print(pm10); Serial.print(",");
Serial.print(methaneValue); Serial.println(";");
delay(2000);
Data Formatting: Constructs and sends a data string to the Serial Monitor in the format required by the ML model: DATA:<sensor values>. The delay(2000); creates a 2-second delay between readings.
3.Reading Prediction from Python Script
// Read prediction from Python script
if (Serial.available() > 0) {
String prediction = Serial.readStringUntil('n');
int separator = prediction.indexOf(',');
String aqiValue = prediction.substring(0, separator);
String aqiCategory = prediction.substring(separator + 1);
// Display prediction on LCD
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("AQI: ");
lcd.print(aqiValue);
lcd.setCursor(0, 1);
lcd.print("Category: ");
lcd.print(aqiCategory);
}
}
Prediction Display: Checks if data is available from the Serial interface. It reads the prediction ( Python script) and splits it into AQI value and category. This information is displayed on the LCD.
// Function to read data from Nova PM sensor
bool readPMData(int &pm1_0, int &pm2_5, int &pm10) {
if (pmsSerial.available() >= 32) {
uint8_t buffer[32];
pmsSerial.readBytes(buffer, 32);
if (buffer[0] == 0x42 && buffer[1] == 0x4D) {
pm1_0 = (buffer[10] << 8) | buffer[11]; // PM 1.0
pm2_5 = (buffer[12] << 8) | buffer[13]; // PM 2.5
pm10 = (buffer[14] << 8) | buffer[15]; // PM 10
return true;
}
}
return false;
}
Functionality: Reads a 32-byte data packet from the PMS5003 sensor. If the packet header is correct (0x42 and 0x4D), it extracts PM values from specific bytes and assigns them to pm2_5, and pm10. Returns true if data is successfully read; otherwise, it returns false.
6. MODEL INTEGRATION CODE EXPLANATION
import serial
import time
import numpy as np
from tensorflow.keras.models import load_model
The script imports necessary libraries: serial for serial communication, time for delays, numpy for data manipulation, and tensorflow.keras.models to load the pre-trained AQI prediction model.
load_model loads a neural network model from the specified file path (6final.h5). This model is trained to predict AQI based on sensor data input from the Maixduino.
ser = serial.Serial('COM15', 115200, timeout=1)
time.sleep(2)
def get_aqi_category(prediction):
if prediction < 50:
return "Healthy"
elif prediction < 100:
return "Moderate"
elif prediction < 150:
return "Unhealthy for Sensitive Groups"
elif prediction < 200:
return "Unhealthy"
elif prediction < 300:
return "Very Unhealthy"
else:
return "Hazardous"
This function categorizes AQI predictions into specific health-related levels, such as "Healthy," "Moderate," and "Unhealthy," based on widely recognized AQI thresholds.
while True:
if ser.in_waiting > 0:
data = ser.readline().decode().strip()
if data.startswith("DATA:"):
try:
values = list(map(int, data[5:-1].split(',')))
if len(values) == 6:
input_data = np.array(values).reshape(1, -1)
aqi_prediction = model.predict(input_data)
aqi = int(aqi_prediction[0][0])
category = get_aqi_category(aqi)
response = f"{aqi},{category}n"
ser.write(response.encode())
print(f"Sent to Maixduino: {response}")
else:
print(f"Received data does not contain 6 values: {data}")
except Exception as e:
print(f"Error processing data: {e}")
time.sleep(2)
The script continuously checks for incoming data from the Maixduino. If available, it reads and decodes the data as a string.
Recognizes data starting with "DATA:" as sensor input. It extracts six sensor values (like those for CO, general air quality, hydrogen sulfide, PM2.5, PM10, and methane) and validates that all six values are present.
The sensor values are converted to a NumPy array and reshaped to match the input shape of the loaded model, which then predicts the AQI.
The predicted AQI is rounded and categorized using get_aqi_category, converting it into readable categories.
The script formats the AQI and category as a response and sends it back to the Maixduino for potential display or further handling.
7.ABOUT OUR TRAINED ML MODEL
Implementation of Predicting Air Quality with Neural Networks
1. Dataset
The model uses the Air Quality Data in India (2015 - 2020), which contains features such as:
PM2.5, PM10, NO, NH3, CO, SO2, O3, and other air pollutants.
2. Data Preprocessing
Missing Data Handling: Checked for and handled missing values in the dataset.
Feature Standardization:
The features were standardized using StandardScaler to ensure all values are on a similar scale, which improves neural network performance.
Data Splitting:
The dataset was split into training and testing sets using train_test_split to evaluate the model’s generalization ability.
3. Exploratory Data Analysis (EDA)
Performed EDA to detect and remove outliers, identify patterns, and ensure data quality, which helps improve the model’s reliability.
4. Model Architecture
A Neural Network was built using TensorFlow's Sequential model, consisting of:
Multiple Dense Layers: These hidden layers have neurons activated using ReLU (Rectified Linear Unit) to introduce non-linearity.
Output Layer: A single neuron in the output layer predicts the continuous air quality value (e.g., AQI or pollutant concentration).
5. Model Compilation & Training
Optimizer: The model was compiled using the Adam optimizer for efficient training.
Loss Function: Mean Squared Error (MSE) was used as the loss function to minimize the difference between predicted and actual values.
Training: The model was trained using TensorFlow's fit() function.
6. Performance Evaluation
Mean Squared Error (MSE) on Test Data: The model achieved an MSE of 100.50 on the test data, indicating the average squared difference between predicted and true values.
8.NOVELTY
The primary novelty of your Maixduino-based AQI monitoring project lies in its combination of real-time air quality monitoring with advanced machine learning predictions, all integrated on compact, low-cost hardware. Here’s a breakdown of its unique features:
Integration of Multiple Environmental Sensors:
Our project gathers data from various environmental sensors (CO, PM2.5, PM10, methane, H₂S, and ozone). This multi-sensor approach provides a more holistic view of air quality compared to typical setups that may only measure a single pollutant.
Real-Time AQI Prediction and Categorization Using a Neural Network Model:
Unlike traditional AQI monitors, which simply display raw sensor data, our project leverages a neural network to predict the AQI and categorize it in real time. The model interprets the combination of multiple pollutants to provide an accurate AQI reading, which helps users understand the overall air quality and its health implications.
Efficient, Low-Cost Hardware Platform with Maixduino:
Implementing this on Maixduino provides an affordable, compact solution without sacrificing processing capabilities. Maixduino’s compatibility with AI and sensor integration makes it ideal for an IoT-based AQI system, which could be deployed in various locations easily, even in cost-sensitive environment.
HARDWARE SETUP
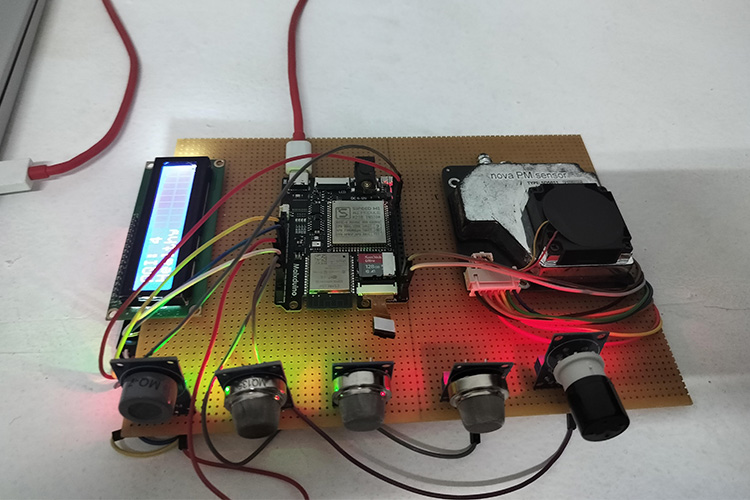
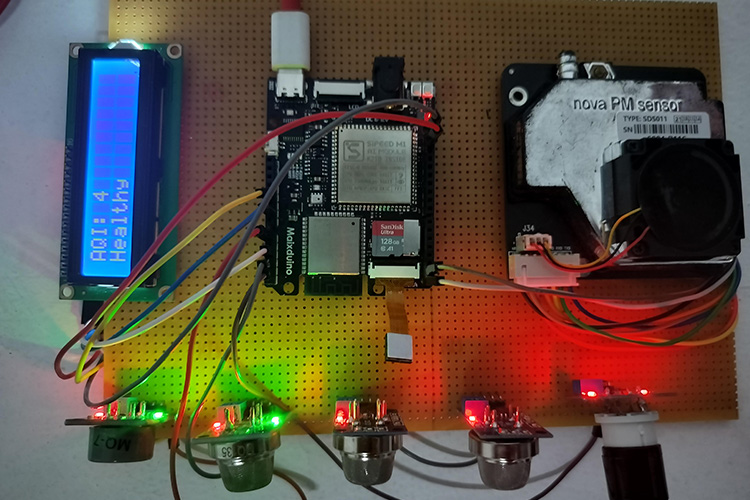
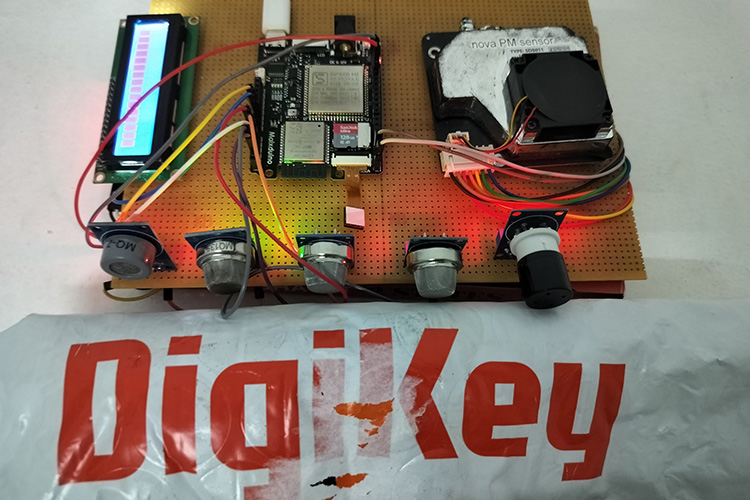
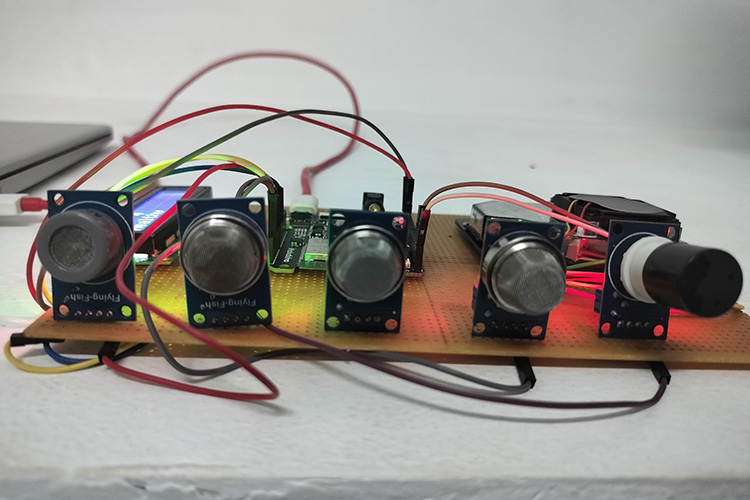
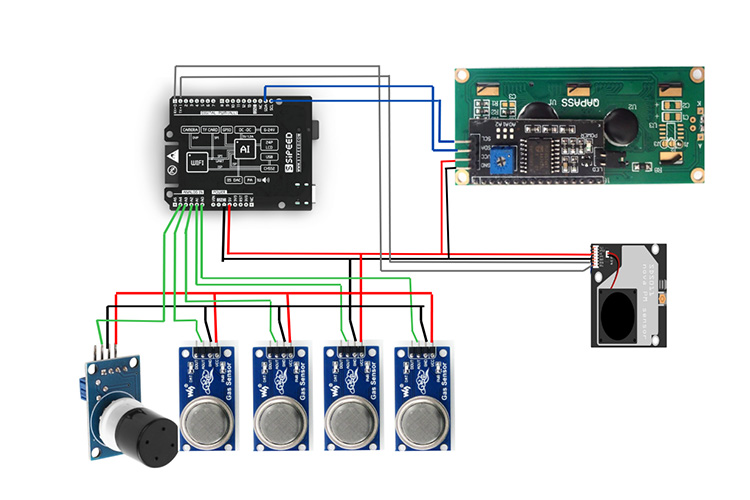