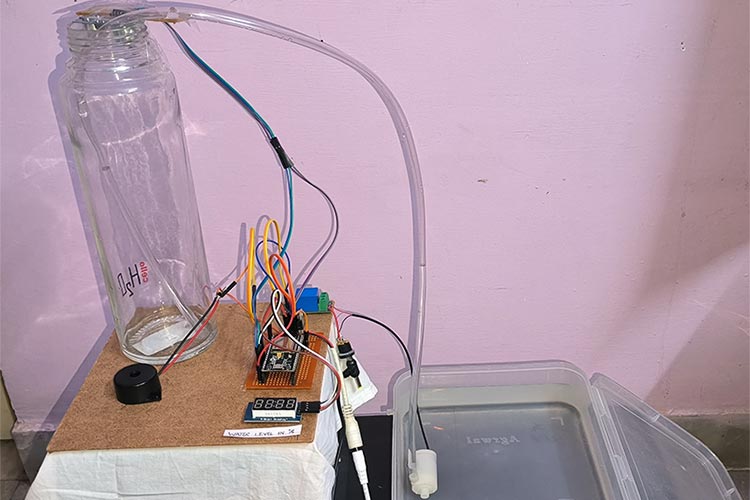
Water wastage due to prolonged pump operation can have significant environmental, financial, and ethical implications. It leads to the unnecessary consumption of a finite resource, water, resulting in both higher costs and environmental harm. By monitoring and reducing water wastage, individuals, businesses, and organizations can conserve water, save money, and contribute to more sustainable and responsible water management practices.
Objective
The project aims to measure the water level inside a tank, display the water level percentage on a 4-digit 7-segment display, and control a pump (or any other water-related equipment) based on the water level. Additionally, it includes a buzzer to provide alerts when the water level reaches critical points.
Components Used
- ESP32 microcontroller
- Ultrasonic sensor (HC-SR04)
- Relay module .
- Buzzer
- TM1637 4-digit 7-segment display
- DC Pump
- LM7805 Voltage Regulator
- 9V Battery with Connector
Circuit Diagram
1. trigPin (GPIO 14) HC-SR04: Connected to the trigger pin of the ultrasonic sensor. It sends a short pulse to trigger the sensor.
2. echoPin (GPIO 12) HC-SR04: Connected to the echo pin of the ultrasonic sensor. It receives the echo signal to measure the time it takes for the sound wave to return.
3. relayPin (GPIO 25): Connected to a relay module to control a pump or other equipment. It is used to turn the relay on (LOW) or off (HIGH) based on the water level percentage.
4. buzzerPin (GPIO 33): Connected to a buzzer for generating sound alerts. It is used to turn the buzzer on (HIGH) or off (LOW) for a specified duration when the water level reaches either 20% or 80%.
5. CLK_PIN (GPIO 26) 4 DIGIT 7 Segment Display: Connected to the CLK (clock) pin of the TM1637 4-digit 7-segment display module. It's used for data communication with the display.
6. DIO_PIN (GPIO 27) 4 DIGIT 7 Segment Display: Connected to the DIO (data input/output) pin of the TM1637 4-digit 7-segment display module. It's used for data communication with the display.
Working
1. Sensing Water Level: An ultrasonic sensor is used to measure the water level inside a tank or reservoir. It emits short ultrasonic pulses, and the time it takes for these pulses to bounce back is recorded. This time measurement is directly related to the distance from the sensor to the water surface.
2. Distance Calculation: The system calculates the distance based on the time measurement. It knows the speed of sound in air, so it can accurately determine the distance from the sensor to the water surface.
3. Percentage Calculation: The calculated distance is then converted into a percentage, representing the water level. For instance, if the distance is relatively short, the water level is high (e.g., 80% full). If the distance is greater, the water level is lower (e.g., 20% low).
4. Visual Display: The project uses a 4-digit 7-segment display to visually represent the water level as a percentage. The display shows the calculated percentage, allowing users to quickly understand the current water level.
5. Pump Control: A relay module is used to control a water pump. The relay's state is determined by the water level percentage:
- If the water level is below 20%, the relay is activated, turning on the pump to fill the tank.
- If the water level rises above 80%, the relay is deactivated, turning off the pump to prevent overfilling.
6. Buzzer Alerts: To provide immediate alerts, a buzzer is added to the system. It is activated when the water level reaches 20% or 80%.
7. Continuous Monitoring: The system continuously monitors the water level and adjusts the pump's operation as needed. This ensures efficient water management, prevents water wastage, and promotes responsible resource usage.
Code Working
1. Library and Pin Definitions: The code begins by including the necessary library, `TM1637Display`, and defining the GPIO pin numbers for various components, including the ultrasonic sensor, relay, and 4-digit 7-segment display and a buzzer .
2. Variable: The code initializes several variables, including `duration` for measuring the time it takes for the ultrasonic pulse to return, `distanceCm` and `distanceInch` for storing the calculated distance, `relay_state` to control the relay state, and `buzzer_state` to control the buzzer state.
3. Setup Function: In the setup function Serial communication is initiated for debugging purposes. GPIO pins for the ultrasonic sensor's trigger and echo pins, relay control, and buzzer control are configured.
The relay is initially set to a HIGH state (off), and the brightness of the 4-digit 7-segment display is set to a medium level (brightness level 5).
4. Loop Function: The loop function continuously performs the following tasks:
- Sends a short pulse from the ultrasonic sensor's trigger pin to initiate a distance measurement.
- Measures the time it takes for the sound wave to return and calculates the distance in centimeters (`distanceCm`).
- Maps the `distanceCm` to a water level percentage based on the provided mapping
- Displays the water level percentage on the 4-digit 7-segment display using the `showNumberDec` function.
- Controls the relay based on the water level percentage:
- If the water level is above 80%, the relay is turned off (HIGH).
- If the water level is at or below 20%, the relay is turned on (LOW).
- Check if the water level percentage is equal to 20 or 80 and, if so, activate the buzzer for 2 seconds before turning it off.
The code works by continuously measuring the water level using an ultrasonic sensor and calculating the water level as a percentage. It displays this percentage on a 4-digit 7-segment display and controls a relay to manage the pump. The buzzer provides audible alerts when the water level reaches critical points. The system is designed to prevent water wastage and optimize water management based on the detected water levels.
#include <TM1637Display.h>
const int trigPin = 14;
const int echoPin = 12;
const int relayPin = 25;
const int buzzerPin = 33;
#define SOUND_SPEED 0.034
#define CM_TO_INCH 0.393701
#define CLK_PIN 26
#define DIO_PIN 27
int relay_state = 0;
int buzzer_state = LOW;
long duration;
float distanceCm;
float distanceInch;
TM1637Display display(CLK_PIN, DIO_PIN);
void setup() {
Serial.begin(115200);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(relayPin, OUTPUT);
pinMode(buzzerPin, OUTPUT);
digitalWrite(relayPin, HIGH);
display.setBrightness(5);
}
void loop() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distanceCm = duration * SOUND_SPEED / 2;
int waterLevelPercentage = map(distanceCm, 3, 20, 100, 20);
Serial.print("Distance (cm): ");
Serial.println(distanceCm);
Serial.print("Water Level Percentage: ");
Serial.println(waterLevelPercentage);
display.showNumberDec(waterLevelPercentage);
if (waterLevelPercentage > 80) {
// statement
digitalWrite(buzzerPin, HIGH);
delay(1500);
digitalWrite(buzzerPin, LOW);
Serial.println("pin high");
digitalWrite(relayPin, HIGH);
} else if (waterLevelPercentage <= 20) {
// statement
digitalWrite(buzzerPin, HIGH);
delay(1500);
digitalWrite(buzzerPin, LOW);
Serial.println("pin low");
digitalWrite(relayPin, LOW);
} else {
// statement
Serial.println("else");
}
delay(1000);
}