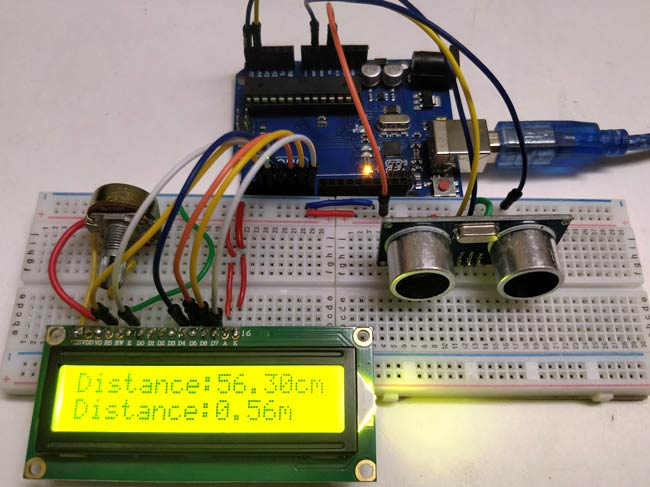
Ultrasonic sensors are great tools to measure distance and detect objects without any actual contact with the physical world. It is used in several applications, like in measuring liquid level, checking proximity and even more popularly in automobiles to assist in self-parking or anti-collision systems. Previously we have also build many Ultrasonic Sensor projects like water level detecting, Ultrasonic Radar etc . This is an efficient way to measure small distances precisely. In this project, we have used the HC-SR04 Ultrasonic Sensor with Arduino to determine the distance of an obstacle from the sensor. The basic principle of ultrasonic distance measurement is based on ECHO. When sound waves are transmitted in the environment then waves return back to the origin as ECHO after striking on the obstacle. So we only need to calculate the traveling time of both sounds means outgoing time and returning time to origin after striking on the obstacle. As the speed of the sound is known to us, after some calculation we can calculate the distance. We are going to use this same technique for this Arduino distance measurement project, so let's get started.
If you are completely new check out this Arduino Ultrasonic Sensor tutorial to learn the basics of ultrasonic sensor and its working. That being said lets get started....
Components Used
- Arduino Uno or Pro Mini
- Ultrasonic sensor Module
- 16x2 LCD
- Scale
- Bread board
- 9 volt battery
- Connecting wires
Ultrasonic Sensor Module
There are many types of Arduino distance sensors, but in this project we have used the HC-SR04 to measure distance in range of 2cm-400cm with an accuracy of 3mm. The sensor module consists of an ultrasonic transmitter, receiver and control circuit. The working principle of ultrasonic sensor is as follows:
- High level signal is sent for 10us using Trigger.
- The module sends eight 40 KHz signals automatically, and then detects whether pulse is received or not.
- If the signal is received, then it is through high level. The time of high duration is the time gap between sending and receiving the signal.
Distance= (Time x Speed of Sound in Air (340 m/s))/2
Timing Diagram
The module works on the natural phenomenon of ECHO of sound. A pulse is sent for about 10us to trigger the module. After which the module automatically sends 8 cycles of 40 KHz ultrasound signal and checks its echo. The signal after striking with an obstacle returns back and is captured by the receiver. Thus the distance of the obstacle from the sensor is simply calculated by the formula given as
Distance= (time x speed)/2.
Here we have divided the product of speed and time by 2 because the time is the total time it took to reach the obstacle and return back. Thus the time to reach obstacle is just half the total time taken.
Ultrasonic Sensor Arduino Circuit Diagram and Explanation
The circuit diagram for arduino and ultrasonic sensor is shown above to measure the distance. In circuit connections Ultrasonic sensor module’s “trigger” and “echo” pins are directly connected to pin 18(A4) and 19(A5) of arduino. A 16x2 LCD is connected with arduino in 4-bit mode. Control pin RS, RW and En are directly connected to arduino pin 2, GND and 3. And data pin D4-D7 is connected to 4, 5, 6 and 7 of arduino.
First of all we need to trigger the ultrasonic sensor module to transmit signal by using arduino and then wait for receive ECHO. Arduino reads the time between triggering and Received ECHO. We know that speed of sound is around 340m/s. so we can calculate distance by using given formula:
Distance= (travel time/2) * speed of sound
Where speed of sound around 340m per second.
A 16x2 LCD is used for displaying distance.
Find more about the working of distance measurement project in this tutorial: Distance measurement using ultrasonic sensor and AVR Microcontroller.
Arduino Ultrasonic Sensor Code for Distance Measurement
The complete code for this ultrasonic distance measurement project is given at the bottom of this page. In the code we read time by using pulseIn(pin). And then perform calculations and displayed result on 16x2 LCD by using appropriate functions.
#include <LiquidCrystal.h>
#define trigger 18
#define echo 19
LiquidCrystal lcd(2,3,4,5,6,7);
float time=0,distance=0;
void setup()
{
lcd.begin(16,2);
pinMode(trigger,OUTPUT);
pinMode(echo,INPUT);
lcd.print(" Ultra sonic");
lcd.setCursor(0,1);
lcd.print("Distance Meter");
delay(2000);
lcd.clear();
lcd.print(" Circuit Digest");
delay(2000);
}
void loop()
{
lcd.clear();
digitalWrite(trigger,LOW);
delayMicroseconds(2);
digitalWrite(trigger,HIGH);
delayMicroseconds(10);
digitalWrite(trigger,LOW);
delayMicroseconds(2);
time=pulseIn(echo,HIGH);
distance=time*340/20000;
lcd.clear();
lcd.print("Distance:");
lcd.print(distance);
lcd.print("cm");
lcd.setCursor(0,1);
lcd.print("Distance:");
lcd.print(distance/100);
lcd.print("m");
delay(1000);
}
Comments
calculation
nice work.. i just have one query
can you tell me the calculation of (voltage change per distance?)
reply me at []
thanks
Arduino.cc
I think you should read the arduino.cc page for more about arduino.
Its Arduino Pro Mini, as
Its Arduino Pro Mini, as mentioned in Components section.
component purchase
can anyone put the link to buy arduino pro mini and IR sensor used here??? plzz help
distance calulation
i need complete detailed calculation of distance
can you program the
can you program the measurement sensor to power a motor at a particular distance for a short period of time
gsm based problem
Hi,
i am also implement this type of projects but real time problem is how to mobile no is store through sms.
and send the sms from gsm your mobile no is registered. second problem tx /rx pin. what pin are using tx and rx . Nobody can entered the this type of sms because sms is tool long .
command:
Tv On tv off
on off
like another message.
after send the sms through the mobile then sms memory is full how to delete the sms memory after gsm read the message . pl use eeprom header and use the eeprom memory in ardunio. then store the mobile no and you can easily change mobile no .
i am already programme the
i am already programme the arduino pro mini using arduino uno board
uno rest to pro mini rest button
uno vcc to pro mini vcc button
uno gnd to pro mini gnd button
uno tx and rx to pro mini txo and rxi button
it is correct or not sir
another one thing is my pro mini board consists
A6 and A7 pin
but in circuit diagram pro mini board consists a A4, A5 pin
it is correct or not
pls tell that sir
You can connect to A6 and A7
You can connect to A6 and A7 but then define the Trigger and 'echo' pin accordingly.
project is success sir thank
project is success sir thank you for your response
but in this programme [340/20000]. how its possible
because in explanation is 340/2
but it is not use in the progromme why sir
Unit Conversion
- Travel time = PulseIn(), gives results in microseconds. This has to be converted to seconds, therefore divide by 1,000,000 (1s = 1000000us).
- The distance measure we want to display in cm instead of m. Therefore, multiply by 1000 (1m =1000cm).
This result in: (340/2)/(1000000)*(1000) = 340/20000
digitalWrite(trigger,LOW);
digitalWrite(trigger,LOW);
delayMicroseconds(2);
digitalWrite(trigger,HIGH);
delayMicroseconds(10);
digitalWrite(trigger,LOW);
delayMicroseconds(2);
pls tell meaning of the lines sir
i don't know sir
Why does this project use
Why does this project use Arduino Pro Mini and all I was seeing from YouTube and other sites are Arduino Mega or Arduino Uno. Is there a significant difference? Does the code above really works?
You can use any model, Code
You can use any model, Code will work in all of them. Further Arduino Pro Mini is small in size and generally used on PCBs because of its size.
Hey if I use ultrasonic
Hey if I use ultrasonic sensor for moving robot then how it will be calculate the distance?????
Check this: Robot using
Check this: Robot using Ultrasonic Sensor and Robot using Arduino
we are making this project
we are making this project and our teacher asked if what is scale?
Scale is used to verifying
Scale is used to verifying the distance, check video.
What should be the possible
What should be the possible program and circuit if centimeter will change to feet?
related ideas
Please give some ideas about projects any concepts you guys know please share it now for final year project
Sanvi there are lots of
Sanvi there are lots of projects here, you can choose anything you like.... Also check the featured projects on the bottom of the page
I’d like to measure human
I’d like to measure human heights in clinic. Is this sensor sensitive to hair? I mean it is important to measure exact height in this case which requires measuring height till the end of skull also to prevent error occurs by hair. I mean height shouldn’t be dependent to hairstyle. can this ultrasonic sensor help me?
No sarath, This sensor will
No sarath, This sensor will be reflected by hair. I cannot think of any sensor that could measure distance past hair.. May be try posting on forum (available on menu on top) and someone might have an answer for you
model of lcd display
hi can you tell me whats the model of lcd display used their?
thanking you
actully its accuracy is very good'and your discreiption and your support made my day thank you ones agian i made some changes for arduino uno
This site is helping a lot
This site is helping a lot for me to easily understand all the arduino related projects and to do it myself.
code error help
im tryn to make a blind stick using arduino nano with buzzer.but there is some error ...can u guyz help me...!
#include <LiquidCrystal.h>
#define trigger 12
#define echo 11
#define BUZZER 10
float time=0,distance=0;
void setup()
{
pinMode(trigger,OUTPUT);
pinMode(echo,INPUT);
pinMode(BUZZER,OUTPUT);
}
void loop()
{
digitalWrite(trigger,LOW);
delayMicroseconds(2);
digitalWrite(trigger,HIGH);
delayMicroseconds(10);
digitalWrite(trigger,LOW);
delayMicroseconds(2);
time=pulseIn(echo,HIGH);*
distance=time*340/20000;
if(distance<40)
{
BUZZER=1;
}
else
{BUZZER=0;}
delay(1000);
}
What error are you getting?
What error are you getting? Also did you look at this project (link below), i think this is what you are looking for
https://circuitdigest.com/microcontroller-projects/arduino-smart-blind-…
ultrasonic arduino distance detector and measurement device
hello, please is it possible to integrate a set of led bulbs to the circuit such that the led bulb comes on with respect to the distance of the obstacle.? if it is possible can someone help me out with the codes?
Yes michael it is possible,
Yes michael it is possible, just interface the LED's to the GPIO pins of the arduino. And add in the program to read the value of distance and make the pins high
aurdino coding in milimeter
hay..i am machanical engg....but interest in electrical & electronic..i see in u tube-ultrasonic ,leaser distance project.. i want know that --can i measured in micron or milimeter in dacimal just like 0.1 mm insteed centimeter..secondry i want measured a wheel center out or runout radial not wheel diameter measure. is this possible to check by ardino project..if yes so please share step by step on my email.id
You cannot measure 0.1mm
You cannot measure 0.1mm using this sensor
Can anyone please tell me the
Can anyone please tell me the cost of project approximately
What changes do I need to
What changes do I need to make for the code if I do not have an LCD and just want the distance recorded on serial monitor
Question about potentiometer
Can u explain me what is the use of Potentiometer when we are using a Arduino uno/Why potentiometer is used inthe circuit??
How To Add Buzzer And LED for
How To Add Buzzer And LED for Limit Distance???
Hello, this projetc isso Good, more no image in failere
Hello, this projetc isso Good, more no image in failere
Can you give me a distance meter flow chart.