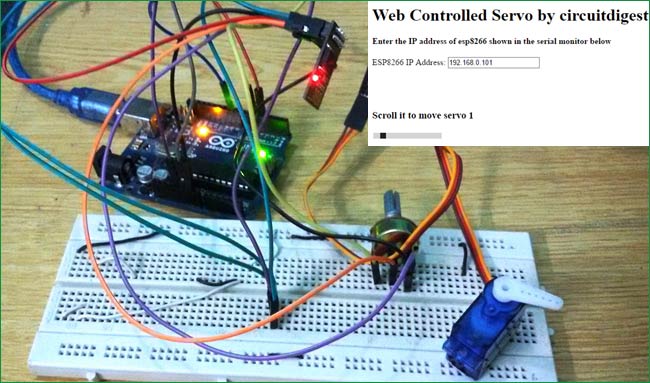
In this post we are going to control a Servo Motor using a web browser with the help of Arduino and Wi-Fi module ESP8266. The ESP8266 will establish a connection between the servo and the web browser through the IP address and then by moving the Slider on the web page, the servo will move accordingly. Even by setting Port Forwarding in your router, you can control the Servo from anywhere in the world over the internet, we will explain that later in this tutorial.
In our previous projects, we have controlled the servo by using many other techniques like we have controlled the servo using Flex sensor, using Force sensor, using MATLAB etc. And we have also covered tutorials on interfacing of Servo with different Microcontrollers like with Arduino, Raspberry Pi, 8051, AVR etc. You can check all Servo related projects here.
Required Components:
The components used in this project are as follows
- Servo (sg90)
- Arduino Uno
- Wi-Fi module ESP8266
- Three 1K resistors
- Connecting wires
Circuit Diagram and Connections:
First of all we will connect the ESP8266 with the Arduino. ESP8266 runs on 3.3V and if you will give it 5V from the Arduino then it won’t work properly and it may get damage. Connect the VCC and the CH_PD to the 3.3V pin of Arduino. The RX pin of ESP8266 works on 3.3V and it will not communicate with the Arduino when we will connect it directly to the Arduino. So, we will have to make a voltage divider for it which will convert the 5V into 3.3V. This can be done by connecting three resistors in series like we did in the circuit. Connect the TX pin of the ESP8266 to the pin 4 of the Arduino and the RX pin of the ESP8266 to the pin 5 of Arduino through the resistors.
ESP8266 Wi-Fi module gives your projects access to Wi-Fi or internet. It is a very cheap device and make your projects very powerful. It can communicate with any microcontroller and it is the most leading devices in the IOT platform. Learn more about using ESP8266 with Arduino here.
Then connect the Servo Motor with the Arduino. The connections of the servo motor with the Arduino are very easier. Connect the VCC and the ground pin of the servo motor to the 5V and the ground of the Arduino and the signal pin of the servo motor to the pin 9 of the Arduino.
How to run it:
To run it, you will have to make an HTML file that will open a web page. For that, you will have to copy below given HTML code (check downwards) and save it in a notepad. There should be ‘.html’ at the end of the file, means file must be saved with ‘.html’ extension. So, if you want to name the file as ‘servo’ then it should be as ‘servo.html’ so that it can be easily get opened in the web browser.
After this, you will have to place the jQuery file in the same folder where you have placed the html file. You can download the HTML file and jQuery from here, extract this zip file and use them directly. jQuery is the library of Java Script which has many JS functions which makes DOM manipulation (Document Object Model), event handling and use of Ajax easy-to-use.
Now open the html file in the web browser, it will look like this.
Now paste the Arduino code in the Arduino IDE and change the name of the Wi-Fi and the password with your Wi-Fi network name and password and upload the code. You will be given with an IP address in the Serial Monitor. Type this IP address in the box on web page. Now when you will move the slider, the Servo will move according to the Slider. So that is how you can control the Servo using Webpage.
HTML Code Explanation:
Here is the complete HTML code for this Web controlled Servo project, we will get through it line by line:
<!DOCTYPE html > <html> <head> <title>Web controlled Servo</title> <script src="jquery.js"></script> </head> <body> <h1> <b> Web Controlled Servo by circuitdigest.com </b> </h1> <h4> <b> Enter the IP address of esp8266 shown in the serial monitor below </b> </h4> <div style="margin: 0; width:500px; height:80px;"> <FORM NAME="form" ACTION="" METHOD="GET"> ESP8266 IP Address: <INPUT TYPE="text" NAME="inputbox" VALUE="192.168.0.9"> </FORM> </div> <h3> Scroll it to move servo 1 </h3> <input type="range" min="20" max="170" onmouseup="servo1(this.value)" /> </body> <script> $.ajaxSetup({timeout:1000}); function servo1(angle) { TextVar = form.inputbox.value; ArduinoVar = "http://" + TextVar + ":80"; $.get( ArduinoVar, { "sr1": angle }) ; {Connection: close}; } </script> </html>
The <!DOCTYPE html > tag will tell the web browser that which version of html we have used to write the html. This should be written at the top. Everything will be written after that.
The code written between the <html> </html> tags will be read by the browser. These are used to tell the web browser that the html code has started from here. The code written out of it will not be displayed on the webpage. The <head> </head> tags are used to define the title, links, jQuery and style. We have defined the title and the jQuery script in it. The data written in the <title></title> will be the name of the tab in the browser. In our code, we have named the tab as the “Web Controlled Servo”.
The <script></script> tags are used to include the jQuery. The jQuery is the JavaScript library and it greatly simplifies our code.
The body tags are used to define all the elements onto the webpage like textbox, range slider, button, text area etc. We can also define sizes for the elements. The h1, h2, h3, h4, h5 and h6 headings are used to write the data in different sizes. In our code, we have used the h1, h3 and h4 headings.
Then we have created a textbox for writing the IP address in it. It will match the IP address with the ESP8266 and will send the data at this IP address. After that, we created a slider (input type="range") that will send the value to the function named “servo1” from angle 20 to 170 for moving the slider.
function servo1(angle) { TextVar = form.inputbox.value; ArduinoVar = "http://" + TextVar + ":80"; $.get( ArduinoVar, { "sr1": angle }) ; {Connection: close}; }
Then the function will be executed and it will send the value to the Arduino to move the servo and will close the connections.
Arduino Code Explanation:
Complete Arduino Code for this Web Controlled Servo is given below in Code section. First of all we will include the Software Serial and the servo libraries. If you don’t have these libraries, then install it before uploading the code. Then define the pins where we have connected the ESP8266.The ‘DEBUG true’ will display the messages of the ESP8266 on the Serial Monitor. Then define the servo pin and declare a variable for servo.
#include <SoftwareSerial.h> #include <Servo.h> SoftwareSerial esp(4, 5); //set ESP8266 RX pin = 5, and TX pin = 4 #define DEBUG true //display ESP8266 messages on Serial Monitor #define servopin 9 //servo 1 on digital port 9 Servo ser; //servo 1
Then tell the Arduino that at which pin we have connected the servo and set it to max position. Then set the baud rate of serial monitor and the ESP8266 at ‘115200’.
ser.attach(servopin); ser.write(maxpos); ser.detach(); Serial.begin(115200); esp.begin(115200);
Below code will connect the ESP8266 to the Wi-Fi of your router which you have entered in the code and will tell you the IP address at which it will communicate with the webpage and will receive the data.
sendData("AT+RST\r\n", 2000, DEBUG); //reset module sendData("AT+CWMODE=1\r\n", 1000, DEBUG); //set station mode sendData("AT+CWJAP=\"Arsenal\",\"12345678\"\r\n", 2000, DEBUG); //connect wifi network while(!esp.find("OK")) { //wait for connection } sendData("AT+CIFSR\r\n", 1000, DEBUG); //show IP address sendData("AT+CIPMUX=1\r\n", 1000, DEBUG); //allow multiple connections sendData("AT+CIPSERVER=1,80\r\n", 1000, DEBUG); //start web server on port 80
In the void loop() function, ESP8266 will check that if the data is arrived or not. If the data is arrived then it will read this data and will show it on the serial monitor and also will move the servo according to this data.
if (esp.available()) //check if there is data available on ESP8266 { if (esp.find("+IPD,")) //if there is a new command { String msg; esp.find("?"); //run cursor until command is found msg = esp.readStringUntil(' '); //read the message String command = msg.substring(0, 3); //command is informed in the first 3 characters "sr1" String valueStr = msg.substring(4); //next 3 characters inform the desired angle int value = valueStr.toInt(); //convert to integer if (DEBUG) { Serial.println(command); Serial.println(value); }
Finally sendData function is used to send the commands to the ESP8266 and to check their response.
String sendData(String command, const int timeout, boolean debug) { String response = ""; esp.print(command); long int time = millis(); while ( (time + timeout) > millis()) { while (esp.available()) { char c = esp.read(); response += c; } } if (debug) { Serial.print(response); } return response; }
We have setup a local server to demonstrate its working, you can check the Video below. But to control the Servo from anywhere, you need to forward the port 80 (used for HTTP or internet) to your local or private IP address (192.168*) of you device. After port forwarding all the incoming connections will be forwarded to this local address and you can just open the given HTML file and enter the public IP address of your internet in the Textbox on webpage. You can forward the port by logging into your router (192.168.1.1) and find the option to setup the port forwarding.
#include <SoftwareSerial.h> //Including the software serial library
#include <Servo.h> //including the servo library
SoftwareSerial esp(4, 5);
#define DEBUG true //This will display the ESP8266 messages on Serial Monitor
#define servopin 9 //connect servo on pin 9
Servo ser; //variable for servo
int current_pos = 170;
int v = 10;
int minpos = 20;
int maxpos = 160;
void setup()
{
ser.attach(servopin);
ser.write(maxpos);
ser.detach();
Serial.begin(115200);
esp.begin(115200);
sendData("AT+RST\r\n", 2000, DEBUG); //This command will reset module
sendData("AT+CWMODE=1\r\n", 1000, DEBUG); //This will set the esp mode as station mode
sendData("AT+CWJAP=\"wifi-name\",\"wifi-password\"\r\n", 2000, DEBUG); //This will connect to wifi network
while(!esp.find("OK")) { //this will wait for connection
}
sendData("AT+CIFSR\r\n", 1000, DEBUG); //This will show IP address on the serial monitor
sendData("AT+CIPMUX=1\r\n", 1000, DEBUG); //This will allow multiple connections
sendData("AT+CIPSERVER=1,80\r\n", 1000, DEBUG); //This will start the web server on port 80
}
void loop()
{
if (esp.available()) //check if there is data available on ESP8266
{
if (esp.find("+IPD,")) //if there is a new command
{
String msg;
esp.find("?"); //run cursor until command is found
msg = esp.readStringUntil(' '); //read the message
String command = msg.substring(0, 3); //command is informed in the first 3 characters "sr1"
String valueStr = msg.substring(4); //next 3 characters inform the desired angle
int value = valueStr.toInt(); //convert to integer
if (DEBUG) {
Serial.println(command);
Serial.println(value);
}
delay(100);
//move servo to desired angle
if(command == "sr1") {
//limit input angle
if (value >= maxpos) {
value = maxpos;
}
if (value <= minpos) {
value = minpos;
}
ser.attach(servopin); //attach servo
while(current_pos != value) {
if (current_pos > value) {
current_pos -= 1;
ser.write(current_pos);
delay(100/v);
}
if (current_pos < value) {
current_pos += 1;
ser.write(current_pos);
delay(100/v);
}
}
ser.detach(); //dettach
}
}
}
}
String sendData(String command, const int timeout, boolean debug)
{
String response = "";
esp.print(command);
long int time = millis();
while ( (time + timeout) > millis())
{
while (esp.available())
{
char c = esp.read();
response += c;
}
}
if (debug)
{
Serial.print(response);
}
return response;
}
Comments
Is it possible to control it from site which is on web server?
Is it possible to control it from site which is on web server? fro example from justexample.com?
garbage
I have run this project, it works fine, but serial monitor shows lot of mixed garbage message along with normal message...also moving the slider to left or right some times ESP8266 responds, sometimes it does not, also on serial monitor many times sr1,20 or sr1,170 is displayed correctly and some times garbage is displayed,,,how can I get rid of this garbage....I tried various baud rates like 9600, 115200, 57600 for Serail.begin and/or esp.begin. Rx pin of ESP8266 is connected to three 1K resistors combination from Tx of Arduino, to adjust for 3.3 v expected at ESP8266 Rx. Can I connect Tx of Arduino directly to Rx of 8266. will it be safe ? will it remove garbage ?
esp8266 and arduino uno
hai team
there are so many pieces of code i am getting confusion in which code i need to dump for esp8266 and arduino uno....please clarify it sir.
Awesome
Thanks for such projects