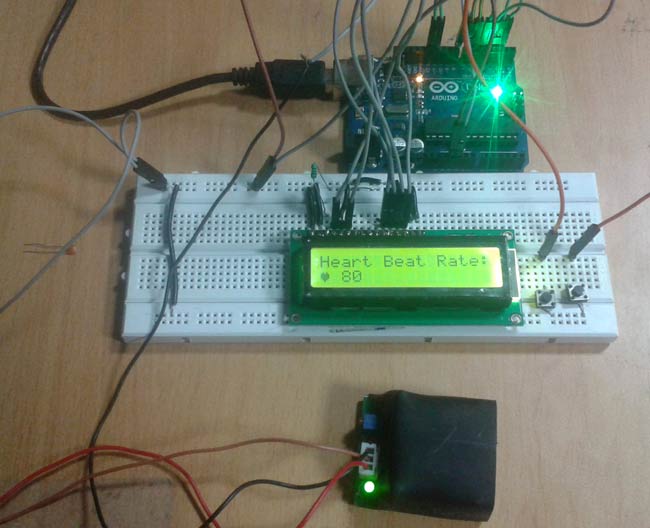
Heart rate, body temperature and blood pressure monitoring are very important parameters of human body. Doctors use various kind of medical apparatus like thermometer for checking fever or body temperature, BP monitor for blood pressure measurement and heart rate monitor for heart rate measurement. In this project, we have built an Arduino based heartbeat monitor which counts the number of heartbeats in a minute. Here we have used a heartbeat sensor module which senses the heartbeat upon putting a finger on the sensor.
Components
- Arduino
- Heart Beat sensor module
- 16x2 LCD
- Push button
- Bread board
- Power
- Connecting wires
Working of Heartbeat Monitor Project
Working of this project is quite easy but a little calculation for calculating heart rate is required. There are several methods for calculating heart rate, but here we have read only five pulses. Then we have calculated total heart beat in a minute by applying the below formula:
Five_pusle_time=time2-time1;
Single_pulse_time= Five_pusle_time /5;
rate=60000/ Single_pulse_time;
where time1 is first pulse counter value
time2 is list pulse counter value
rate is final heart rate.
When first pulse comes, we start counter by using timer counter function in arduino that is millis();. And take first pulse counter value form millis();. Then we wait for five pulses. After getting five pulses we again take counter value in time2 and then we substarct time1 from time2 to take original time taken by five pulses. And then divide this time by 5 times for getting single pulse time. Now we have time for single pulse and we can easily find the pulse in one minute, deviding 600000 ms by single pulse time.
Rate= 600000/single pulse time.
In this project we have used Heart beat sensor module to detect Heart Beat. This sensor module contains an IR pair which actually detect heart beat from blood. Heart pumps the blood in body which is called heart beat, when it happens the blood concentration in body changes. And we use this change to make a voltage or pulse electrically.
Circuit Diagram and Explanation
Circuit of heartbeat monitor is shown below, which contains arduino uno, heart beat sensor module, reset button and LCD. Arduino controls whole the process of system like reading pulses form Heart beat sensor module, calculating heart rate and sending this data to LCD. We can set the sensitivity of this sensor module by inbuilt potentiometer placed on this module.
Heart beat sensor module’s output pin is directly connected to pin 8 of arduino. Vcc and GND are connected to Vcc and GND. A 16x2 LCD is connected with arduino in 4-bit mode. Control pin RS, RW and En are directly connected to arduino pin 12, GND and 11. And data pin D4-D7 is connected to pins 5, 4, 3 and 2 of arduino. And one push button is added for resetting reading and another is used to start the system for reading pulses. When we need to count heart rate, we press start button then arduino start counting pulses and also start counter for five seconds. This start push button is connected to pin 7 and reset push button is connected to pin 6 of arduino with respect to ground.
Program Description
In code we have used digital read function to read output of Heart Beat sensor module and millis() fuction for calculating time and then calculate Heart Rate.
Before this we have initiazed all the components that we used in this project.
and here we have pullup the push button line by using software pullup.
#include<LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
int in = 8;
int Reset=6;
int start=7;
int count=0,i=0,k=0,rate=0;
unsigned long time2,time1;
unsigned long time;
byte heart[8] =
{
0b00000,
0b01010,
0b11111,
0b11111,
0b11111,
0b01110,
0b00100,
0b00000
};
void setup()
{
lcd.createChar(1, heart);
lcd.begin(16,2);
lcd.print("Heart Beat ");
lcd.write(1);
lcd.setCursor(0,1);
lcd.print("Monitering");
pinMode(in, INPUT);
pinMode(Reset, INPUT);
pinMode(start, INPUT);
digitalWrite(Reset, HIGH);
digitalWrite(start, HIGH);
delay(1000);
}
void loop()
{
if(!(digitalRead(start)))
{
k=0;
lcd.clear();
lcd.print("Please wait.......");
while(k<5)
{
if(digitalRead(in))
{
if(k==0)
time1=millis();
k++;
while(digitalRead(in));
}
}
time2=millis();
rate=time2-time1;
rate=rate/5;
rate=60000/rate;
lcd.clear();
lcd.print("Heart Beat Rate:");
lcd.setCursor(0,1);
lcd.print(rate);
lcd.print(" ");
lcd.write(1);
k=0;
rate=0;
}
if(!digitalRead(Reset))
{
rate=0;
lcd.clear();
lcd.print("Heart Beat Rate:");
lcd.setCursor(0,1);
lcd.write(1);
lcd.print(rate);
k=0;
}
}
Comments
heart beat monitoring with temperature sensor and LCD display
good morning. i have a project heart beat monitoring including temperature sensor and the heart beat reading should be appear on the LCD also the temperature. i saw your code it is the complete code for the heart beat rate? can you help me on my project? i use LM34 temperature sensor.
thanks
Hello Lorenz ! I also have
Hello Lorenz ! I also have the same project of preparing a system which has both temperature sensing module and heart rate measuring .... Can you please send me details of your project ...
enquiry about project
I am doing my final year project on Heart rate monitor based on arduino. Could you please send me the complete project information along with code and circuit to my mail[], it will be very helpful. Please do send the project that u have along with temparature sensor interface.
Could you please share the project full files
Bro i"m also interested in doing the same project of heart beat monitoring system
it would be a great help if you share the project details to me
hi..lorenzo, even i'm
hi..lorenzo, even i'm planning to do my project based on heart rate monitor and temparature sensor..Can u please help me with the project with clear picture of the circuit and extra code for the temperature sensor.
Thanks in adv
bro if you have the circuit
bro if you have the circuit and code of heart rate monitor and temparature sensor plz help me by sending it to me plz im in need of it the same is the project of mine
Its a simple Heartbeat Sensor
Its a simple Heartbeat Sensor Module, any Heartbeat sensor should work here.
arduino program on rf transmiter
pls give me reference of program on rf transmiter on arduino sir
Future implementation
My final year project is blood pressure measurement so can we find blood pressure from heart rate plz help me its argent
Y don't v try in msp 430 if
Y don't v try in msp 430 if so how the connection will be
Yes, it can be built using
Yes, it can be built using MSP430, please try to build and share with us.
heart beat reg
Can i know exactly which sensor module is used in this project ??
Can i have the details about the sensor module
This is a 5v Heartbeat sensor
This is a 5v Heartbeat sensor module, you can just purchase it by its name i.e. Pulse sensor or Heartbeat sensor module.
whether it is ok for this .
whether it is ok for this ..the part number of sensor ky039 heart beat sensor
yes, you can use KY-039 heart
yes, you can use KY-039 heart beat sensor.
Can you give specifications
Can you give specifications of power and connecting wires
you can use 12 volt power
you can use 12 volt power adaptor to supply whole circuit at Arduino Board's DC Jack or at pin Vin.
please dont try to connect 12 volt elsewhere in circuit. otherwise you circuit may damage.
Can I use Digital Heart beat
Can I use Digital Heart beat sensor for Atmel Pic Arduino Raspberry Pi
yes you can use heart beat
yes you can use heart beat sensor module for any microcontroller.
can you have same code for
can you have same code for atmega32 i,m making same project
Hi Abhishek,
Hi Abhishek,
do you have any sample code for testing heart beat sensor ij arduino which you used in this project. i am having same sensor i ported everything i had troubleshoot it.but i cant read the value.i want to check the sensor value via serial monitor.whether this sensor read the value or not.could you please share the code for testing heart beat sensor.
Check this link, it has
Check this link, it has testing script with serial monitor, for Arduino.
quiet urgent
I did everything same..but I didn't get proper pulse..getting value is over exceeding .like most of the time I am getting above 100 values..is there any mistake from me?could you please give me the soultion for me?
can you send the name of heart sensors and its code
can you send the name of heart sensors and its code which are using by you and name the pressure sensors using adrino
<p>You can use any Heart beat
You can use any Heart beat sensor module, Read all of the above comments. Full code is already given. And for pressure sensor check this Pressure Sensor BMP180 Interfacing with Arduino Uno
i want full coding for heart
i want full coding for heart rate monitor
code not working
i have connected all the wires and its showing the reading but not accurate reading sometimes its show 340 sometimes 1000 and even sometimes -1 so its not accurate even i adjust the pot of sensor
same problem, i think the
same problem, i think the pulse sensor with analog output dont work with this code, my bpm show 20000, 15000, -5536 :)))
this is my problem , too .
this is my problem , too . please help me .
what i have to do to resolve this???
Heart beat
how do not need to press the button after 5 seconds, press once every ro run forever?
Query about easy pulse sensor V1.1
I need library for this easy pulse sensor V1.1 to run my code and receive data efficiently , share link or send direct on my email thanks
sensor unit
can you provide internal circuit diagram of sensor unit
can i have the complete code
can i have the complete code please.
Check this project for
Check this project for interfacing RF pair with Arduino
heart beat sensor
hello, everyone,...
I wanna ask if pin8 mode is output or input
connect with wifi and android
hi. .
Its a fabulous work of you.
My question is how can we develop this system to monitor (say 5 family members at the same time) heart beat in real time using a web server or with an android device. Well, that just an idea. Please excuse my poor english.
Cheers,
Handy Mosey
The model no of ur heartbeat
The model no of ur heartbeat module ???????
This is a 5v Heartbeat sensor
This is a 5v Heartbeat sensor module, you can just purchase it by its name i.e. Pulse sensor or Heartbeat sensor module. Any Heartbeat sensor should work here.
Monitoring the pulse rate continuously
Can you supply the code for monitoring the pulse continuously? i.e. each pulse is to be taken into consideration and obtain the time bet next pulse and display the rate. Also time in milli seconds can be exported along with pulse rate. This will be very good gazed for measuring HRV. Thanks and awaiting your reply
hi, i have connected all the
hi, i have connected all the wires but i am not getting the correct output, i am getting some random values as output,and i am not using lcd i am checking the output in aurdino serial monitor. should i change anything in code????
help me with arduio code Arduino Based Heartbeat Monitor
Hi bro please,
can I have the full code please...
can you suggest different
can you suggest different model of 16 x 2 lcd display other than LM016L? Because i'm using a different model of lcd display and it does not display any results. thank you.
Any 16x2 LCD should work here
Any 16x2 LCD should work here, you just need to connect the Pins properly.
Hi, i have connected all the
Hi, i have connected all the wires but i am not getting the correct output, i am getting some random values as output,and i am not using lcd i am checking the output in aurdino serial monitor. What should i change in code????can u please give me the code part without LCD interface....
You need to use 16x2 LCD with
You need to use 16x2 LCD with the Given code.
How will the code look like
How will the code look like if we add abnormal beat feature and extend the project with a GSM/GPS SIM 808?
Can't work
Why my project cannot display the heart rate although i get the program and circuit correctly?
i connected everything
i connected everything correctly even though i am not getting the out put correctly. can you please give me the code for arduino serial port.instead of photo diode i am using photo transistor am not getting the correct output.
About code
The arduino doesn't calculate the heart hate thr is any problems in code
NOT GETTING CORRECT OUTPUT
I am using KY-039 Heartbeat sensor and I have connected all the connections properly.
But it is displaying heartbeat rate : -1.
Are their any mistakes in code if so mail me the code please.
Do we need to connect S pin of sensor to 8 pin of arduino and middlepin of sensor to VCC of arduino?
I am not able to understand how to use heartbeat sensor.
At what angle IR LED should be placed?
Someone reply quickly.
regarding calculation
sir while finding rate why u r considering 60000
heartbeat sensor arduino project
Hello fellow Ardweeners..I have completed this project and can offer anyone help if needed..One of the problems that I had was getting the sensors to read an actual heartbeat. It is very tricky and difficult to get your finger in the perfect position that the sensors can read...be patient yet determined you will get it. Use a cell phone camera to make sure the infrared led is emitting, you will see a purple-ish glow, through the camera but not with just your eyes..Once I connected the arduino and started to read the heartbeats I noticed i would always get a number at least 2 times the actual number, ie.. instead of a b.p.of 55 the lcd screen would read 110.I found out that the arduino was counting the small signal at the beginning of the heartbeat as a heartbeat itself, so I simply changed the formula for rate by dividing it by 2...you see on an oscilliscope the heart beat actually appears as 2 pulses in 1 where the beginning of the beat there is a small pulse ,I supposes one of the heart ventricles open followed by a bigger more significant pulse the actual heartbeat.Although not necessary I also added a amplifier to the circuit to increase the gain of the heartbeat, also added a red led as an extra light source for the infrared phototransistor as in actual oximeters there are 2 lights sources both visible and infrared
my email is beatlessteve at gmail dot com..good luck yall!..
I am currently doing design
I am currently doing design project,so my chosen project is heartbeat and temperature monitor.I would like to use arduino for this project but I am not familiar with it .I would like you to pls explain a bit to me about it
Heart Beat Sensor module libary
i want to add KY-039 heart beat sensor. Library in Proteus 8
Any Possible Enhancements to this current Project
Can anyone, please suggest any kind of enhancement possible for this subject. thank you
You can use it as a "Heart
You can use it as a "Heart attack detector", use GSM or wifi to alert the Doctor with a message, when the heart beats goes very high.It will be a great IoT project.
What is the complexity level
What is the complexity level of this project??
Is it difficult to handle the heart rate monitor???
It's a very simple and useful
It's a very simple and useful monitor. I just have one question about the code:
k++;
>>>> while(digitalRead(in));
}
What does this while do? I didn't get it because the pin will be reading zero every time you take a signal from the sensor, so would it get a 1 (HIGH) to continue the loop?
Thanks in advance.
while(digitalRead(in)); will
while(digitalRead(in)); will interrupt the code until the output from the Heart beat sensor becomes zero.
about code
sir its output stays on please wait...
what should i do????
hy can any one please tell .
hy can any one please tell . i got problem when i presss the button lcd shows "please wait" and remains there and does not show any value
I m not using Display. And i
I m not using Display. And i want to print actual data ie actual heart rate of the human body. so how can i achieve this? For actual data representation what is hanges in this program.
How can i send the message
How can i send the message ( heart rate ) to a doctor or a hospital unit using GSM in interafacing with the arduino...plz send me the circuit & code..if you know
i got one problem ,when i
i got one problem ,when i placed any object like pen on hear beat sensor it dispalys value. pls give solution to this problem
code is not working
i have connected it but it is always showing please wait message .Can anyone help to sort out this problem
heart rate monitor
Bro i am having same problem lcd shows please wait and and stays as it is.what to do now
i neend full code
Please help I can't see the full code of this project. Because of overlap in starting codes please full code. From starting.
1.can we detect whether a
1.can we detect whether a person is prone to heartattack by measuring few samples from time to time?
2.if there is a sudden change in heartbeat....can we generate a warning?
Not getting proper heartbeat sensor output
Hii Sir,
We are using Heartbeat sensor in our project. We done with same type of connection what you given above. I am getting d output, but it is showing 200, 500,600 sometimes -10. I tried many times. But not getting d exact output. Sir please help me. Because we need to submit our project by Wednesday. Please give me solution within Tuesday .
In the circuit diagram, there
In the circuit diagram, there is a resistance what is the value of this one ?
can I have the full code please...